Android App Development Interview Questions to Help You Ace Any Interview : Are you aspiring to be an Android app developer? Whether you’re a fresh graduate looking for your first job or an experienced developer aiming to switch roles, preparing for an Android app development interview is crucial. In this article, we’ve compiled a list of essential Android app development interview questions to help you ace your next interview. From fundamental concepts to more advanced topics, we’ve got you covered. Let’s dive in!
Scope of Android – Android Interview Questions
Android plays a vital role in the mobile market as it enables smartphone users to download and access various applications that offer a wide range of services. Android developers have the opportunity to publish their apps on different platforms such as SlideME, Mobango, Amazon App Store, Opera Mobile Store, and more. Many of these platforms are free, contributing to the extensive market for Android mobile application development, particularly in regions like India.
Advantages & Disadvantages of Android
Advantages of Android
- Open-source: Android is an open-source platform, which means there are no licensing fees, development costs, or distribution charges for developers.
- Platform Independence: Android development tools, like Android Studio and Android SDK, are platform-independent, allowing developers to create Android applications on various operating systems.
- Highly Optimized Virtual Machine: Android utilizes a highly optimized virtual machine called the Dalvik Virtual Machine (DVM), which was later replaced by the Android RunTime (ART) virtual machine. ART was introduced with Android Lollipop 5.0 (API level 21) to execute Android apps more efficiently.
- Support for Various Technologies: Android supports a wide range of technologies, including camera, speech recognition, Bluetooth, Wi-Fi, EDGE, and more.
- Abundance of Apps: The Google Play Store and alternative stores offer millions of Android applications, providing users with a vast selection of apps to choose from.
Disadvantages of Android – Android Interview Questions
- Fake Applications: The presence of numerous fake applications in the market poses a risk as some may attempt to steal user data.
- Fragmentation: Android devices come in various screen sizes and dimensions, and more critically, they run different versions of the Android operating system. This fragmentation can lead to compatibility issues, where an app that runs smoothly on one version may encounter problems on another.
- Version Compatibility: Developing apps that are compatible with multiple Android versions can be challenging. This is because new Android versions may introduce changes or deprecate features, requiring developers to adapt their apps to ensure they work correctly across various OS versions.
- Background Processes: In older Android versions, many applications run continuously in the background, impacting device performance and battery life. Android 8.0 and later versions have imposed restrictions on background processes to address this issue.
- Data Usage: The multitude of background processes on Android devices can result in high data consumption, potentially leading to slower internet speeds and draining the device’s battery quickly.
1. What is Android and why is it important?
Answer: Android is an open-source operating system primarily designed for mobile devices, developed by Google. It’s important because it powers billions of Smartphone’s and other devices worldwide, making it a significant platform for app development. Its open nature, vast user base, and extensive developer community make it a lucrative space for developers.
2. Explain the Android app components.
Answer: Android app components are essential building blocks of an Android application. The main components are:
- Activities: Represent user interfaces and screen interactions.
- Services: Perform background tasks independently of the UI.
- Broadcast Receivers: Respond to system or app-wide events.
- Content Providers: Manage data sharing between apps.
3. What is the AndroidManifest.xml file used for?
Answer: The AndroidManifest.xml file is a crucial configuration file for Android apps. It contains essential information about the app, such as permissions required, activities, services, and broadcast receivers used, ensuring the Android system understands how to run the app.
4. What is the activity lifecycle, and why is it important to understand?
Answer: The activity lifecycle consists of various states (e.g., onCreate, onStart, onResume, onPause, onStop, onDestroy) that an activity goes through during its lifetime. Understanding it is essential to manage resources, handle user interactions, and maintain a smooth user experience as an app transitions between states.
5. Differentiate Activities from Services.
Answer: Activities and Services in Android serve different purposes:
Activities:
- Activities represent the user interface and the screen that users interact with.
- They typically have a UI, interact with the user, and are often associated with user actions like tapping buttons or entering text.
- Activities have a lifecycle, and they can transition through states like onCreate, onStart, onResume, onPause, onStop, and onDestroy.
- Activities are primarily used for creating the user interface and handling user interactions.
Services:
- Services, on the other hand, are background components that perform tasks without a user interface.
- They are often used for long-running operations, such as downloading files, playing music, or processing data in the background.
- Services have their lifecycle methods but are typically less focused on user interactions.
- They can run even if the app’s user interface (activity) is not visible.
In short, Activities are for user interfaces and interactions, while Services are for background tasks and operations.
6. What is an Adapter in Android?
In Android, an adapter serves as a crucial intermediary connecting an AdapterView (like a ListView, GridView, or Spinner) with the underlying data source. The adapter plays a pivotal role in managing and providing access to the data for the AdapterView. It essentially acts as a data bridge.
The adapter stores the data and efficiently delivers it to the AdapterView as needed. The AdapterView, in turn, uses this data to populate and display various UI elements, allowing users to interact with the data seamlessly. This enables the creation of dynamic and interactive user interfaces in Android applications, such as scrollable lists or dropdown menus.
7. What is Android Debug Bridge(ADB)?
Answer: The Android Debug Bridge (ADB) is a command-line tool that enables developers to establish communication with an emulator instance. It provides the capability for developers to remotely execute shell commands, facilitating the deployment and execution of applications on an emulator.
8. What is DDMS?
DDMS(Dalvik Debug Monitor Server) is a debugging tool in the Android platform. It gives the following list of debugging features:
- Port forwarding services.
- Thread and heap information.
- Logcat.
- Screen capture on the device.
- Network traffic tracking.
- Incoming call and SMS spoofing.
- Location data spoofing.
9. What is the life cycle of Android activity?
- Answer: OnCreate(): Triggered during activity creation. Here, views are set up, and data is collected from bundles.
- OnStart(): Invoked when the activity is becoming visible to the user. It may be followed by onResume() when the activity comes to the foreground, or onStop() when it goes into the background.
- OnResume(): Called as the activity initiates interaction with the user.
- OnPause(): This method is triggered when the activity is transitioning to the background but hasn’t been terminated.
- OnStop(): Executed when the activity is no longer visible to the user.
- OnDestroy(): Invoked when the activity is either completed or destroyed.
- OnRestart(): Occurs after the activity has been halted, just before it restarts.
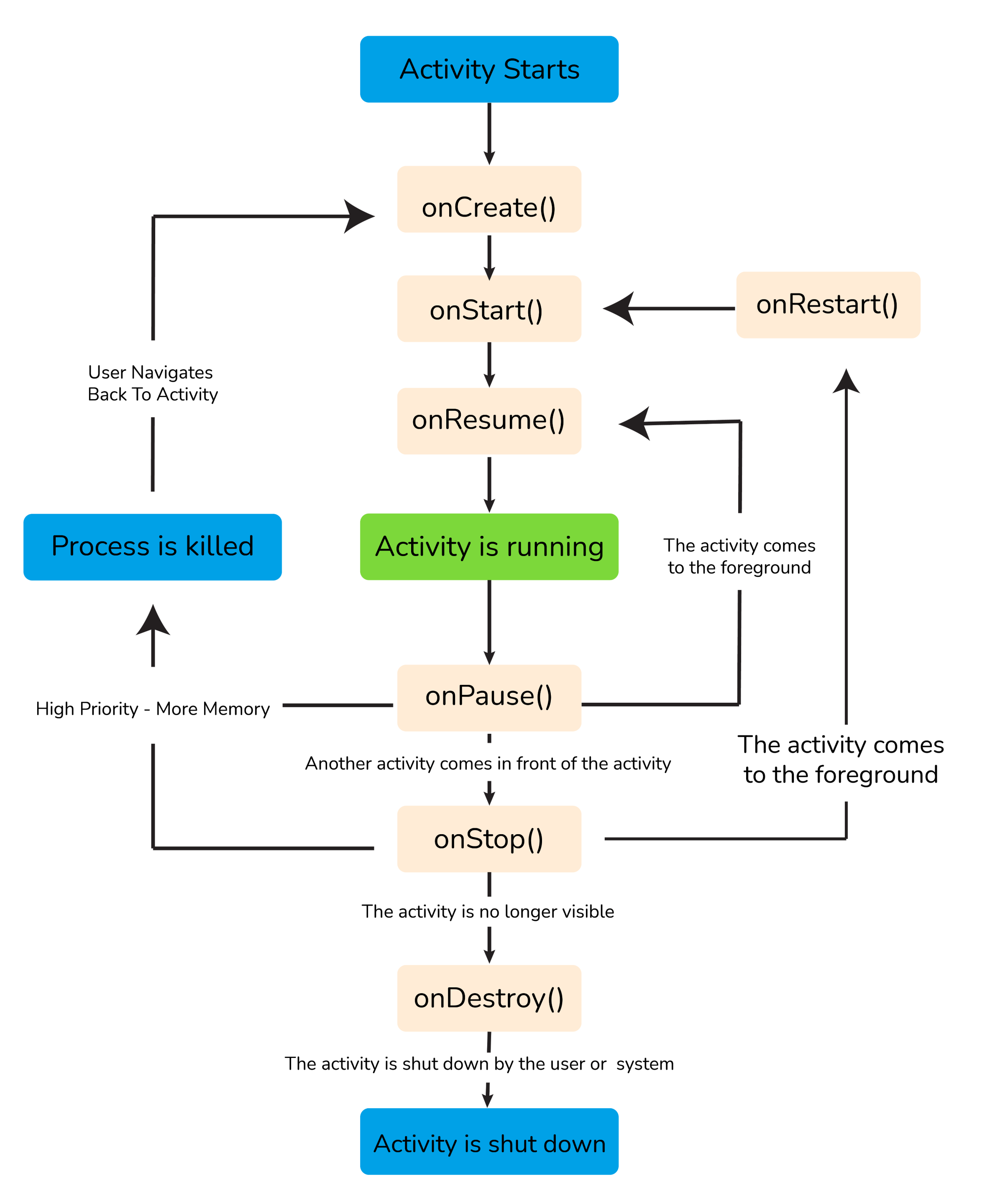
10. What is the difference between Serializable and Parcelable?
Answer: Serializable and Parcelable are used for object serialization in Android. Serializable is a Java interface used for basic object serialization, while Parcelable is a more efficient Android-specific mechanism for passing objects between components, especially when performance matters.
11. How does the Android memory management system work?
Answer: Android uses a combination of heap and stack memory. The heap stores objects and data, and the stack keeps track of method calls and local variables. Android also employs a garbage collector to reclaim memory from objects no longer in use.
12. What is the importance of the AsyncTask class in Android?
Answer: AsyncTask is used to perform background tasks in Android, such as network operations, without blocking the UI thread. It’s essential for maintaining a responsive user interface. However, in modern Android development, alternatives like Kotlin Coroutines and RxJava are preferred for better concurrency handling.
13. What is the ViewHolder pattern, and why is it used in RecyclerView?
Answer: The ViewHolder pattern is used to improve the performance of RecyclerView by recycling views. It caches references to view elements within an item layout, reducing the need to repeatedly call findViewById(), which can be costly.
14. What is Dependency Injection in Android, and how does it improve code quality?
Answer: Dependency Injection (DI) is a design pattern used to inject dependencies (e.g., objects, services) into classes rather than having them create their own dependencies. It improves code quality by making classes more modular, testable, and less tightly coupled, which leads to easier maintenance and testing.
15. What are the best practices for optimizing Android app performance?
Answer: Best practices for optimizing Android app performance include using background threads for time-consuming tasks, optimizing layouts with ConstraintLayout, reducing memory usage by efficiently loading and caching images, and monitoring performance with tools like the Android Profiler.
16. What is Service in Android?
Answer: Services are components that can run in the background independently of the UI. They are suitable for long-running tasks, such as playing music or downloading large files. However, you need to manage threading yourself to prevent blocking the main UI thread.
17. What is the concept of ‘Intent’ in the context of Android, and could you explain the different types of Intents available in Android?
Answer: In Android, an Intent is a fundamental component that facilitates communication between different parts of an application or between different Android applications. It is an abstract description of an operation to be performed. Intents are used for various purposes, such as starting activities, services, or broadcasting events.
There are two main types of Intents in Android:
Explicit Intents and Implicit Intents
Explicit Intents:
- Explicit Intents are used when you have a specific target component (activity, service, or broadcast receiver) in mind that you want to launch or interact with.
- They include the component name (e.g., the fully qualified class name of an activity) explicitly in the Intent.
- Explicit Intents are typically used for within-app navigation or when you know the exact component you want to start.
Example of an explicit Intent to start a specific activity:
Intent intent = new Intent(MainActivity.this, SecondActivity.class); startActivity(intent);
Implicit Intents:
- Implicit Intents are more flexible and are used when you want to perform an action, and you don’t specify a particular component. Instead, you declare the action you want to perform, and the Android system decides which component should handle that action.
- They typically include information such as action, data, and category.
- Implicit Intents are often used for actions like opening a web page, sharing content, or selecting an image from the device’s gallery.
Example of an implicit Intent to open a web page:
Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse("https://www.example.com")); startActivity(intent);
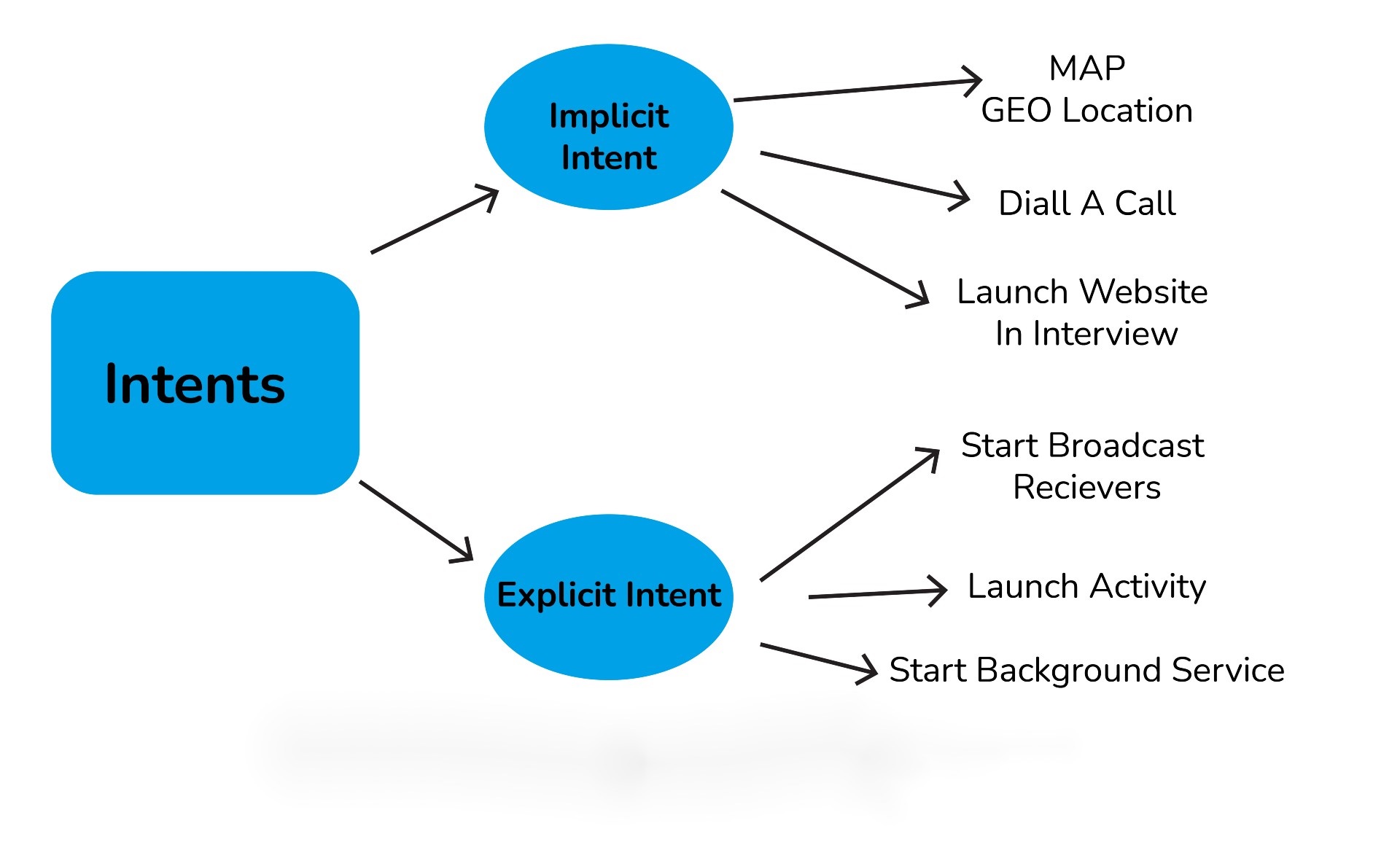
In addition to these two categories are also:
Pending Intents:
-
- Pending Intents are a special type of Intent used in situations where you want to perform an action at a later time, or from a different context.
- They are often used with features like notifications, alarms, and services. You can think of them as “Intents to be executed later.”
Broadcast Intents:
-
- Broadcast Intents are used to send system-wide messages or notifications to other apps or components.
- They are typically used with Broadcast Receivers to listen for and respond to system events or custom events within an app.
18. How can you handle background tasks efficiently in Android?
Discuss different approaches like Services, WorkManager, and JobScheduler for managing background tasks and their use cases.
Answer: In Android, there are several ways to handle background tasks efficiently, each with its use cases:
- Services: Services are components that can run in the background independently of the UI. They are suitable for long-running tasks, such as playing music or downloading large files. However, you need to manage threading yourself to prevent blocking the main UI thread.
- WorkManager: WorkManager is a part of Android Jetpack and is designed for deferrable and reliable background tasks. It’s great for tasks like syncing data, sending notifications, or performing periodic work. WorkManager handles task execution efficiently, even in scenarios where the app is not actively running.
- JobScheduler: JobScheduler is a system service that allows you to schedule tasks based on specific conditions, such as network connectivity or device charging status. It’s useful for tasks that need optimization based on system conditions and for conserving battery life.
19. What is ProGuard, and how does it enhance Android app security?
Explain how ProGuard can be used to obfuscate and shrink the code to protect an Android app from reverse engineering.
Answer: ProGuard is a code shrinking and obfuscation tool that enhances the security of Android apps. It works by making the app’s bytecode more difficult to understand and reverse engineer. Here’s how it enhances security:
- Code Obfuscation: ProGuard renames classes, methods, and variables to names that are meaningless to human readers. This makes it significantly more challenging for reverse engineers to understand the app’s logic.
- Code Shrinking: ProGuard removes unused code and resources, reducing the size of the APK. Smaller APKs are harder to reverse engineer because they contain fewer clues about the app’s functionality.
- Optimization: ProGuard performs code optimization to make the app run faster and more efficiently, which can also make reverse engineering more challenging.
By using ProGuard, developers can make it more difficult for attackers to reverse engineer their apps and extract sensitive information or create malicious versions of the app.
20. Describe the Android app signing process. Outline the steps involved in signing an Android app and the importance of app signing for security and distribution.
Answer: The Android app signing process involves adding a digital signature to your app’s APK (Android Package) file. This signature is crucial for both security and distribution purposes. Here are the steps involved:
- Generate a Keystore: You create a Keystore file that contains a private key and certificate. This is typically done during app development and is used to sign all versions of your app.
- Sign the App: During the build process, your app is signed with the private key from the Keystore. This creates a signed APK.
- Distribute the Signed APK: The signed APK can now be distributed to users through app stores or other distribution channels. The digital signature ensures the integrity of the APK.
- Verification on Installation: When a user installs the app, the Android system checks the app’s signature against the one in the Keystore. If they match, the app is considered genuine and safe to install.
The importance of app signing includes:
- Security: Signing ensures that the app hasn’t been tampered with or altered since it was signed. Users can trust that the app hasn’t been modified by malicious actors.
- App Updates: To release updates to your app, you must sign them with the same Keystore used for the original version. This guarantees a seamless update process.
- Distribution: App stores and platforms require signed APKs for distribution. Without a valid signature, your app won’t be accepted.
21. What are the differences between an Intent and a Bundle? Differentiate between Intents and Bundles and explain when to use each for passing data between Android components.
Answer: Intents and Bundles are both used for passing data between Android components, but they serve different purposes:
- Intent:
- Purpose: Intents are primarily used for communication between different components, such as starting activities, services, or broadcasting events.
- Data Types: Intents can carry a wide range of data types, including primitive types, Parcelable objects, and even URIs.
- Use Cases: Use Intents to initiate actions like opening a new activity, starting a service, or broadcasting an event to multiple components.
- Bundle:
- Purpose: Bundles are used for passing data within the same component or between components within the same process.
- Data Types: Bundles are flexible and can hold various data types, including primitive types, Parcelable objects, and Serializable objects.
- Use Cases: Use Bundles when you need to pass data between different parts of the same app, such as between fragments in an activity or between an activity and its associated service.
In summary, Intents are used for inter-component communication, often involving different app components or even external apps, while Bundles are employed for intra-component data transfer within the same app’s components
22. What is the AndroidX library, and how does it simplify Android development? Provide an overview of AndroidX and its role in providing backward-compatible libraries for Android app development.
Answer: AndroidX is a set of libraries provided by Google to simplify Android app development. It plays a crucial role in providing backward-compatible libraries to ensure that apps work seamlessly on different Android versions and devices. Here’s how AndroidX simplifies Android development:
- Backward Compatibility: AndroidX replaces the older Android Support Library and provides a consistent set of libraries that are compatible with older Android versions. This allows developers to use modern Android features even on older devices.
- Modularity: AndroidX libraries are modular, meaning you can include only the specific components you need, reducing the size of your app and avoiding unnecessary dependencies.
- Improved Architecture: AndroidX includes libraries like LiveData, ViewModel, and Room that promote modern app architecture patterns like MVVM and make it easier to write clean and maintainable code.
- Consistency: AndroidX ensures a consistent look and behavior of UI components across different Android versions, reducing fragmentation-related issues.
- Jetpack: AndroidX is a part of Android Jetpack, which is a suite of libraries, tools, and guidance for Android app development. Jetpack components help with tasks like navigation, data storage, and UI design.